下面這些C語言基礎演算法案例都是經過測試和驗證過了的,歡迎各位使用。
本文是該系列的第一篇,都是一些相對初級的演算法,很適合剛開始學C語言的同學。
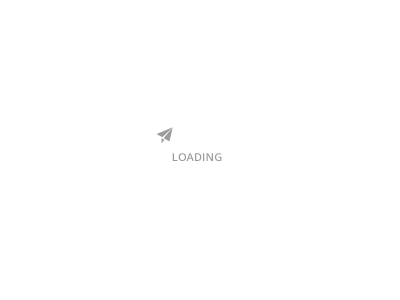
1、C語言列印一條語句
原始碼:
/* C Program to print a sentence。 */#include int main(){ printf(“C Programming”); /* printf() prints the content inside quotation */ return 0;}
輸出:
C Programming
2、C語言列印使用者輸入的一個整數
原始碼:
#include int main(){ int num; printf(“Enter a integer: ”); scanf(“%d”, &num); /* Storing a integer entered by user in variable num */ printf(“You entered: %d”,num); return 0;}
輸出:
Enter a integer: 25You entered: 25
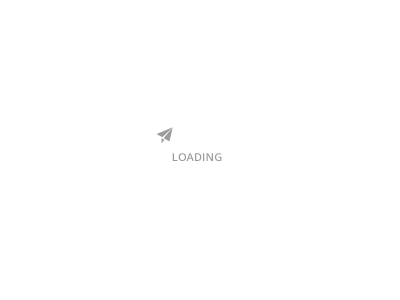
3、C語言實現兩個整數相加
原始碼:
/*C programming source code to add and display the sum of two integers entered by user */#include int main( ){ int num1, num2, sum; printf(“Enter two integers: ”); scanf(“%d %d”, &num1, &num2); /* Stores the two integer entered by user in variable num1 and num2 */ sum = num1 + num2; /* Performs addition and stores it in variable sum */ printf(“Sum: %d”, sum); /* Displays sum */ return 0;}
輸出:
Enter two integers: 1211Sum: 23
4、C語言實現兩個小數相乘
原始碼:
/*C program to multiply and display the product of two floating point numbers entered by user。 */#include int main( ){ float num1, num2, product; printf(“Enter two numbers: ”); scanf(“%f %f”, &num1, &num2); /* Stores the two floating point numbers entered by user in variable num1 and num2 respectively */ product = num1 * num2; /* Performs multiplication and stores it */ printf(“Product: %f”, product); return 0;}
輸出:
Enter two numbers: 2。41。1Product: 2。640000
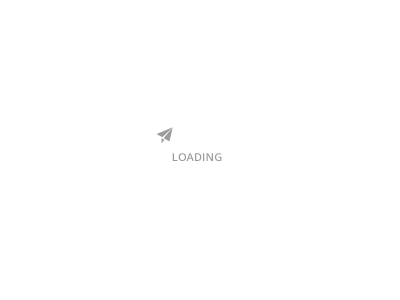
學習從來不是一個人的事情,要有個相互監督的夥伴,工作需要學習C/C++或者為了入行、轉行學習C/C++的夥伴可以私信回覆小編“學習”領取全套免費C/C++學習資料、影片
你想成為一個什麼樣的程式設計師?
5、C語言查詢字元的ASCII值
原始碼:
/* Source code to find ASCII value of a character entered by user */#include int main(){ char c; printf(“Enter a character: ”); scanf(“%c”, &c); /* Takes a character from user */ printf(“ASCII value of %c = %d”, c, c); return 0;}
輸出:
Enter a character: GASCII value of G = 71
6、C語言根據使用者輸入的整數做商和餘數
原始碼:
/* C Program to compute remainder and quotient */#include int main(){ int dividend, divisor, quotient, remainder; printf(“Enter dividend: ”); scanf(“%d”, ÷nd); printf(“Enter divisor: ”); scanf(“%d”, &divisor); quotient = dividend / divisor; /* Computes quotient */ remainder = dividend % divisor; /* Computes remainder */ printf(“Quotient = %d\n”, quotient); printf(“Remainder = %d”, remainder); return 0;}
輸出:
Enter dividend: 25Enter divisor: 4Quotient = 6Remainder = 1
7、C語言獲取整型、單精度浮點型、雙精度浮點型和字元型的長度
基本語法是:
temp = sizeof(operand);/* Here, temp is a variable of type integer, i。e, sizeof() operator returns integer value。 */
原始碼:
/* This program computes the size of variable using sizeof operator。 */#include int main(){ int a; float b; double c; char d; printf(“Size of int: %d bytes\n”, sizeof(a)); printf(“Size of float: %d bytes\n”, sizeof(b)); printf(“Size of double: %d bytes\n”, sizeof(c)); printf(“Size of char: %d byte\n”, sizeof(d)); return 0;}
輸出:
Size of int: 4 bytesSize of float: 4 bytesSize of double: 8 bytesSize of char: 1 byte
注:可能會由於系統的不同出來的結果也不盡相同。
8、C語言獲取關鍵字long的長度範圍
原始碼:
#include int main(){ int a; long int b; /* int is optional。 */ long long int c; /* int is optional。 */ printf(“Size of int = %d bytes\n”, sizeof(a)); printf(“Size of long int = %ld bytes\n”, sizeof(b)); printf(“Size of long long int = %ld bytes”, sizeof(c)); return 0;}
輸出:
Size of int = 4 bytesSize of long int = 4 bytesSize of long long int = 8 bytes
9、C語言交換數值
原始碼:
#include int main(){ float a, b, temp; printf(“Enter value of a: ”); scanf(“%f”,&a); printf(“Enter value of b: ”); scanf(“%f”, &b); temp = a; /* Value of a is stored in variable temp */ a = b; /* Value of b is stored in variable a */ b = temp; /* Value of temp(which contains initial value of a) is stored in variable b*/ printf(“\nAfter swapping, value of a = %。2f\n”, a); printf(“After swapping, value of b = %。2f”, b); return 0;}
輸出:
Enter value of a: 1。20Enter value of b: 2。45After swapping, value of a = 2。45After swapping, value of b = 1。2
10、C語言檢查數值是奇數還是偶數
原始碼:
/*C program to check whether a number entered by user is even or odd。 */#include int main(){ int num; printf(“Enter an integer you want to check: ”); scanf(“%d”, &num); if((num % 2) == 0) /* Checking whether remainder is 0 or not。 */ printf(“%d is even。”, num); else printf(“%d is odd。”, num); return 0;}
輸出1:
Enter an integer you want to check: 2525 is odd。
輸出2:
Enter an integer you want to check: 1212 is even。
也可以用條件運算子解決:
/* C program to check whether an integer is odd or even using conditional operator */#include int main(){ int num; printf(“Enter an integer you want to check: ”); scanf(“%d”, &num); ((num%2) == 0) ? printf(“%d is even。”, num) : printf(“%d is odd。”, num); return 0;}
11、C語言檢查是母音還是子音
原始碼:
#include int main(){ char c; printf(“Enter an alphabet: ”); scanf(“%c”, &c); if(c == ‘a’ || c == ‘A’ || c == ‘e’ || c == ‘E’ || c == ‘i’ || c == ‘I’ || c == ‘o’ || c == ‘O’ || c == ‘u’ || c == ‘U’) printf(“%c is a vowel。”, c); else printf(“%c is a consonant。”, c); return 0;}
輸出1:
Enter an alphabet: ii is a vowel。
輸出2:
Enter an alphabet: GG is a consonant。
也可以用條件運算子解決
/* C program to check whether a character is vowel or consonant using conditional operator */#include int main(){ char c; printf(“Enter an alphabet: ”); scanf(“%c”, &c); (c == ‘a’ || c == ‘A’ || c == ‘e’ || c == ‘E’ || c== ‘i’ || c== ‘I’ || c == ‘o’ || c == ‘O’ || c == ‘u’ || c == ‘U’) ? printf(“%c is a vowel。”, c) : printf(“%c is a consonant。”, c); return 0;}
輸出結果和上面的程式相同。
12、C語言實現從三個數值中查詢最大值
實現1:
/* C program to find largest number using if statement only */#include int main(){ float a, b, c; printf(“Enter three numbers: ”); scanf(“%f %f %f”, &a, &b, &c); if (a > =b && a >= c) printf(“Largest number = %。2f”, a); if (b >= a && b >= c) printf(“Largest number = %。2f”, b); if (c >= a && c >= b) printf(“Largest number = %。2f”, c); return 0;}
實現2:
/* C program to find largest number using if。。。else statement */#include int main(){ float a, b, c; printf(“Enter three numbers: ”); scanf(“%f %f %f”, &a, &b, &c); if (a >= b) { if(a >= c) printf(“Largest number = %。2f”, a); else printf(“Largest number = %。2f”, c); } else { if(b >= c) printf(“Largest number = %。2f”, b); else printf(“Largest number = %。2f”, c); } return 0;}
實現3:
/* C Program to find largest number using nested if。。。else statement */#include int main(){ float a, b, c; printf(“Enter three numbers: ”); scanf(“%f %f %f”, &a, &b, &c); if(a >= b && a >= c) printf(“Largest number = %。2f”, a); else if(b>=a && b>=c) printf(“Largest number = %。2f”, b); else printf(“Largest number = %。2f”, c); return 0;}
輸出結果相同:
Enter three numbers: 12。213。45210。193Largest number = 13。45
13、C語言解一元二次方程
原始碼:
/* Program to find roots of a quadratic equation when coefficients are entered by user。 *//* Library function sqrt() computes the square root。 */#include #include
輸出1:
Enter coefficients a, b and c: 2。345。6Roots are: -0。87+1。30i and -0。87-1。30i
輸出2:
Enter coefficients a, b and c: 410Roots are: 0。00 and -0。25
14、C語言檢查是否是閏年
原始碼:
/* C program to check whether a year is leap year or not using if else statement。 */#include int main(){ int year; printf(“Enter a year: ”); scanf(“%d”, &year); if(year%4 == 0) { if( year%100 == 0) /* Checking for a century year */ { if ( year%400 == 0) printf(“%d is a leap year。”, year); else printf(“%d is not a leap year。”, year); } else printf(“%d is a leap year。”, year); } else printf(“%d is not a leap year。”, year); return 0;}
輸出1:
Enter year: 20002000 is a leap year。
輸出2:
Enter year: 19001900 is not a leap year。
輸出3:
Enter year: 20122012 is a leap year。
15、C語言檢查一個數是正數、負數還是零
原始碼:
#include int main(){ float num; printf(“Enter a number: ”); scanf(“%f”, &num); if (num <= 0) { if (num == 0) printf(“You entered zero。”); else printf(“%。2f is negative。”, num); } else printf(“%。2f is positive。”, num); return 0;}
也可以使用if-else語句
/* C programming code to check whether a number is negative or positive or zero using nested if。。。else statement。 */#include int main(){ float num; printf(“Enter a number: ”); scanf(“%f”, &num); if (num<0) /* Checking whether num is less than 0*/ printf(“%。2f is negative。”, num); else if (num>0) /* Checking whether num is greater than zero*/ printf(“%。2f is positive。”, num); else printf(“You entered zero。”); return 0;}
輸出1:
Enter a number: 12。312。30 is positive。
輸出2:
Enter a number: -12。3-12。30 is negative。
輸出3:
Enter a number: 0You entered zero。
16、C語言檢查某字串是不是字母
原始碼:
/* C programming code to check whether a character is alphabet or not。 */#include int main(){ char c; printf(“Enter a character: ”); scanf(“%c”,&c); if( (c >= ‘a’&& c <= ‘z’) || (c >= ‘A’ && c <= ‘Z’)) printf(“%c is an alphabet。”, c); else printf(“%c is not an alphabet。”, c); return 0;}
輸出1:
Enter a character: ** is not an alphabet
輸出2:
Enter a character: KK is an alphabet
17、C語言計算自然數的和
原始碼:
/* This program is solved using while loop。 */#include int main(){ int n, count, sum = 0; printf(“Enter an integer: ”); scanf(“%d”, &n); count = 1; while(count <= n) /* while loop terminates if count>n */ { sum += count; /* sum=sum+count */ ++count; } printf(“Sum = %d”, sum); return 0;}
也可以使用for迴圈語句
/* This program is solve using for loop。 */#include int main(){ int n, count, sum=0; printf(“Enter an integer: ”); scanf(“%d”, &n); for (count = 1; count <= n; ++count) /* for loop terminates if count>n */ { sum += count; /* sum=sum+count */ } printf(“Sum = %d”, sum); return 0;}
輸出:
Enter an integer: 100Sum = 5050
也可以透過if-else語句
/* This program displays error message when user enters negative number or 0 and displays the sum of natural numbers if user enters positive number。 */#include int main(){ int n, count, sum=0; printf(“Enter an integer: ”); scanf(“%d”, &n); if (n <= 0) printf(“Error!!!!”); else { for(count = 1; count <= n; ++count) /* for loop terminates if count>n */ { sum += count; /* sum=sum+count */ } printf(“Sum = %d”, sum); } return 0;}
18、C語言計算階乘
對於任意正數n,階乘指的是:
factorial = 1*2*3*4。。。。n
如果數值是負數,那麼階乘就不存在。並且我們規定,0的階乘就是1。
原始碼:
/* C program to display factorial of an integer if user enters non-negative integer。 */#include int main(){ int n, count; unsigned long long int factorial = 1; printf(“Enter an integer: ”); scanf(“%d”, &n); if (n < 0) printf(“Error!!! Factorial of negative number doesn‘t exist。”); else { for (count = 1; count <= n; ++count) /* for loop terminates if count>n */ { factorial *= count; /* factorial=factorial*count */ } printf(“Factorial = %lu”, factorial); } return 0;}
輸出1:
Enter an integer: -5Error!!! Factorial of negative number doesn’t exist。
輸出2:
Enter an integer: 10Factorial = 3628800